Captchas are the tool to determine whether the user is a real user or a bot(automated user), captcha provides challenges to users like calculating the sum, finding specific images, etc.. it is difficult for a bot to solve a random challenge but easy for humans. so let’s start to react to ReCaptcha implantations.
in this post, we learn how to implement google ReCaptcha V2 in react, which is owned and maintained by Google, we will use the react-google-recaptcha npm package for implementation.
You can download the working example of the ReCaptcha component https://github.com/technostuf/react-google-recaptcha-v2
- Create a React application
- Generating keys for google ReCaptcha
- Install react-google-recaptcha library
- Add code to the file
- Run a React application
Create a React application
Create a React app using the below command.
npx create-react-app react-google-recaptcha-v2
The above command will create a new folder with the app name so you need to move into that folder, use the below command to change the directory.
cd react-google-recaptcha-v2
Generating keys for google ReCaptcha
Open the https://www.google.com/recaptcha/admin/ The captcha registration will look like this
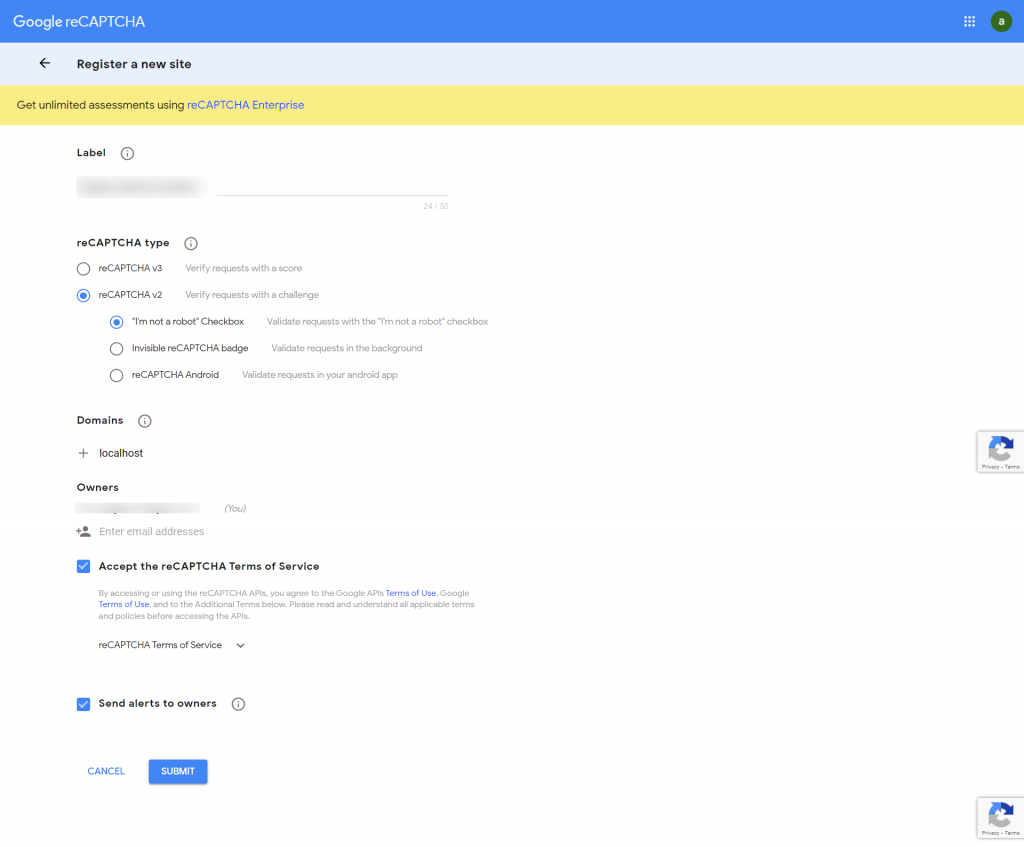
Select the ReCaptcha type V2, because this tutorial will explain for the reCAPTCHA V2, Fill out the rest of the form and submit the form like domain, and label name. After submitting the form you will redirect to the next page where you can find the Site Key and Secret keys for google Recaptcha.
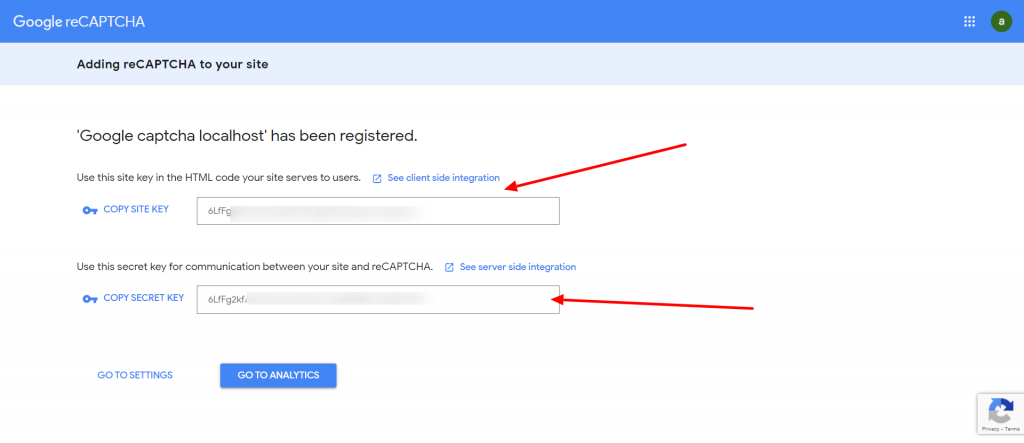
Copy the Site Key by clicking on the COPY SITE KEY link, key will be copied to the clipboard
Install react-google-recaptcha library
We will use the react-google-recaptcha npm package for the implement captcha, so open your terminal window and run the below command
npm i react-google-recaptcha --save
Add code to the file
Create a component file ContactFormComponent.js for the contact form, in this example, I am just explaining about captcha so I have put code for captcha only.
import React from "react";
import ReCAPTCHA from "react-google-recaptcha";
const ContactFormComponent = () => {
let onChange = (value) => {
console.log("Captcha value:", value);
}
return (
<div>
<ReCAPTCHA
sitekey="replace copied site key here"
onChange={onChange}
/>
</div>
)
}
export default ContactFormComponent;
In the above code, onChange will call when the user submits the captcha to google, captcha value should be validated on the backend side when the user submits the form. Below will use to verify on the server-side
let captchaToken = req.body.captchaToken;
// Call Google's API to get score
const res = await axios.post(
`https://www.google.com/recaptcha/api/siteverify?secret=${YOUR_PRIVATE_KEY}&response=${captchaToken}`
);
// Extract result from the API response
if (res.data.success){
console.log('Valid');
} else {
console.log('Invalid');
}
Finally, you have to import ContactFormComponent into App.js
import './App.css';
import ContactFormComponent from './ContactFormComponent';
function App() {
return (
<div className="App">
<ContactFormComponent />
</div>
);
}
export default App;
Run a React application
Run the React application using the following command.
npm start
After compilation, the program opens your browser and runs http://localhost:3000/
Output

You can download the working example of Recaptcha.
https://github.com/technostuf/react-google-recaptcha-v2
Related Post
- React Material UI Form example
- React Material UI Autocomplete with getting selected value
- React Area chart using recharts example
- React Pie chart using recharts with legend and custom label
- React google maps draggable marker example
- React datepicker using the most popular react-datepicker library
- React toast notification using react-toastify with example
- React responsive carousel slider with react-slick
- React tooltip using rc-tooltip with example
- React hook form schema validation using yup