In this article, We will learn how to copy the text to the clipboard in ReactJS using the popular npm package react-copy-to-clipboard so let me explain step by step.
You can download/clone the working example of the copy to the clipboard Github – Copy to Clipboard Example
- Create a React application
- Install react-bootstrap npm package
- Install copy to the clipboard npm package
- Add code to the file
- Run a React application
Create a React application
Create a React app using the below command.
npx create-react-app react-copy-to-clipboard-demo
// if you are using create-react-app (5.0.1) or more than use below command
create-react-app react-copy-to-clipboard-demo
The above command will create a new folder with the app name so you need to move into that folder, use the below command to change the directory.
cd react-copy-to-clipboard-demo
Install react-bootstrap library
We are using bootstrap to design the HTML page, it’s not required to install bootstrap to use copy to clipboard future. the following command will install react-bootstrap and bootstrap package into the application.
npm install --save react-bootstrap bootstrap
OR using yarn
yarn add react-bootstrap bootstrap
Install copy to the clipboard npm package
We will use react-copy-to-clipboard package to copy text, open the terminal and run the following command.
npm i --save react-copy-to-clipboard
Add code to the file
Create a component file CopyToClipboardComponent.js to hold the copy to clipboard code, then we need to import it into App.js.
import React, { useState, useRef } from "react";
import "bootstrap/dist/css/bootstrap.css";
import { CopyToClipboard } from "react-copy-to-clipboard";
const CopyToClipboardComponent = () => {
const [text, setText] = useState("");
const [isCopied, setIsCopied] = useState(false);
const copyToClip = () => {
setIsCopied(true);
setTimeout(() => {
setIsCopied(false);
}, 1000);
};
return (
<>
<div className="container">
<div class="row d-flex justify-content-center text-center">
<h1>React copy to clipboard - technostuf.com</h1>
<hr />
<div className="col-md-8">
<input type="text" value={text} placeholder="Type text here" onChange={(event) => setText(event.target.value)} />
</div>
<div className="col-md-8">
<CopyToClipboard text={text} onCopy={copyToClip}>
<div className="copy-btn">
<button>Copy to Clipboard</button>
{isCopied &&
<span>Text Copied!</span>
}
</div>
</CopyToClipboard>
</div>
</div>
</div>
</>
)
}
export default CopyToClipboardComponent;
Finally, you have to import both files into App.js
import './App.css';
import CopyToClipboardComponent from './CopyToClipboardComponent';
function App() {
return (
<div className="App">
<CopyToClipboardComponent />
</div>
);
}
export default App;
Run a React application
Run the React application using the following command.
npm start
After compilation, the program opens your browser and runs http://localhost:3000/
Output
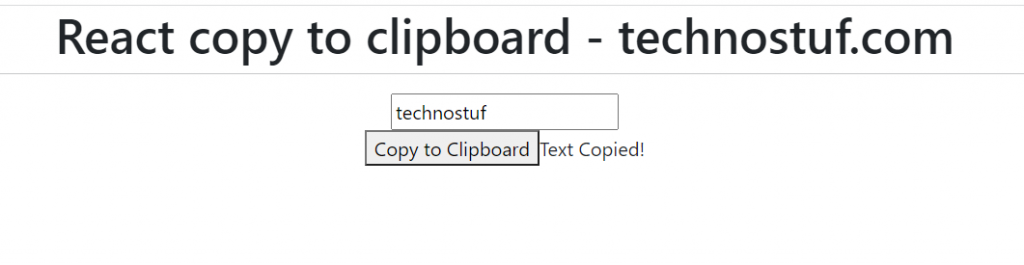
You can download/clone the working example of a copy to the clipboard component.
Github – Copy to Clipboard Example
Related Post
- React Material UI Form example
- React Material UI Autocomplete with getting selected value
- React Area chart using recharts example
- React Pie chart using recharts with legend and custom label
- React google maps draggable marker example
- React datepicker using the most popular react-datepicker library
- React toast notification using react-toastify with example
- React responsive carousel slider with react-slick
- React tooltip using rc-tooltip with example
- React hook form schema validation using yup