React slick is a carousel component built with React. build upon the slick carousel. React slick is used to create caroused slider in a web application, it is easy to use, we can create a responsive image slider, HTML content slider, swipe to slide slider, and many other features supported by the slick slider. today in this post you will learn to create a responsive slider(carousel) using react-slick.
You can download the working example of a react responsive carousel slider https://github.com/technostuf/react-slick-demo
Create a React application
Create a React app using the below command.
npx create-react-app react-swipeble-demo
The above command will create a new folder with the app name so you need to move into that folder, use the below command to change the directory.
cd react-swipeble-demo
Install react-slick library
After creating react app we need to install react-slick from npm or yarn. this package build using a slick carousel
npm install react-slick --save
OR
yarn add react-slick
After installing the react-slick package you need to install CSS in our project
npm install slick-carousel --save
Add code to the file
Add the react carousel code SwipeSlider.js file, We have created a separate file to demonstrate the carousel demo.
import React, { useEffect } from "react";
import Slider from "react-slick";
import "slick-carousel/slick/slick.css";
import "slick-carousel/slick/slick-theme.css";
const SwipeSlider = () => {
const settings = {
infinite: true,
slidesToShow: 3,
lazyLoad: true,
dots: true,
swipeToSlide: true,
responsive: [
{
breakpoint: 1024,
settings: {
slidesToShow: 3,
slidesToScroll: 3,
infinite: true,
dots: true
}
},
{
breakpoint: 600,
settings: {
slidesToShow: 2,
slidesToScroll: 2,
initialSlide: 2
}
},
{
breakpoint: 480,
settings: {
slidesToShow: 1,
slidesToScroll: 1
}
}
],
afterChange: function (index) {
console.log(
`Slider Changed to: ${index + 1}, background: #222; color: #bada55`
);
}
};
const data = [
{
image: "https://i.picsum.photos/id/1015/6000/4000.jpg?hmac=aHjb0fRa1t14DTIEBcoC12c5rAXOSwnVlaA5ujxPQ0I",
caption: "First Slide",
},
{
image: "https://i.picsum.photos/id/100/2500/1656.jpg?hmac=gWyN-7ZB32rkAjMhKXQgdHOIBRHyTSgzuOK6U0vXb1w",
caption: "Second Slide",
},
{
image: "https://i.picsum.photos/id/1025/4951/3301.jpg?hmac=_aGh5AtoOChip_iaMo8ZvvytfEojcgqbCH7dzaz-H8Y",
caption: "Third Slide",
},
{
image: "https://i.picsum.photos/id/1002/4312/2868.jpg?hmac=5LlLE-NY9oMnmIQp7ms6IfdvSUQOzP_O3DPMWmyNxwo",
caption: "Fourth Slide",
},
{
image: "https://i.picsum.photos/id/101/2621/1747.jpg?hmac=cu15YGotS0gIYdBbR1he5NtBLZAAY6aIY5AbORRAngs",
caption: "Fifth Slide",
}
]
return (
<div className="container">
<div class="row d-flex justify-content-center text-center">
<h1 className="w-100 text-center mb-5">React Swipe to Slide using react-slick - technostuf.com</h1>
<hr />
<div className="col-md-8">
<Slider {...settings}>
{data.map((slide, i) => {
return (
<div>
<img
className="d-block w-100"
src={slide.image}
alt={slide.caption}
/>
</div>
)
})}
</Slider>
</div>
</div>
</div >
)
}
export default SwipeSlider;
Useful Props
Right now explaining only used props, you can check the full list of props here
- infinite: Infinitely wrap around contents, Default value is true
- slidesToShow: How many slides to show in the slider at a time, Default value is 1
- lazyLoad: Load the images or content on demand
- infinite: Infinitely wrap around contents, Default value is true
- slidesToShow: How many slides to show in the slider at a time, Default value is 1
- lazyLoad: Load the images or content on demand
- dots: Show a dots in the slider
- swipeToSlide: Enable swipe the slide
- responsive: Customize the slider layout view based on breakpoints (Please check the demo for a better understanding)
- afterChange: Slide change callback
Finally, you have to import both files into App.js.
import './App.css';
import SwipeSlider from './SwipeSlider';
function App() {
return (
<div className="App">
<SwipeSlider />
</div>
);
}
export default App;
Run a React application
Run the React application using the following command.
npm start
After compilation, the program opens your browser and runs http://localhost:3000/
Output
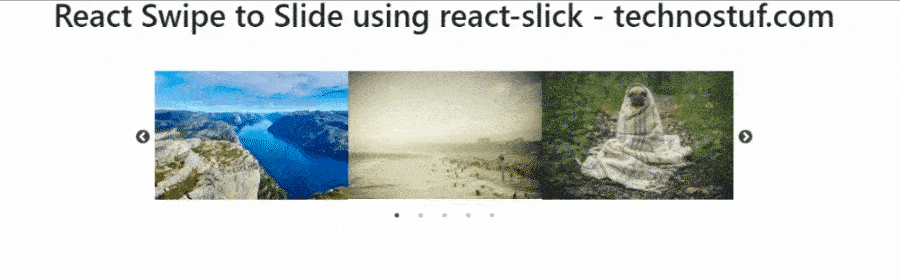
You can download/clone the working example for react responsive carousel slider.
https://github.com/technostuf/react-slick-demo
Related Post
- React Material UI Form example
- React Material UI Autocomplete with getting selected value
- React Area chart using recharts example
- React Pie chart using recharts with legend and custom label
- React google maps draggable marker example
- React datepicker using the most popular react-datepicker library
- React toast notification using react-toastify with example
- React responsive carousel slider with react-slick
- React tooltip using rc-tooltip with example
- React hook form schema validation using yup