Reactjs file upload example; File uploading is an upload file, documents, etc.. from the local computer, mobile, in this tutorial we will learn how to upload a file in reactjs
You can download the working example of a ReactJS file upload https://github.com/technostuf/reactjs-file-upload-example
- Create a React application
- Install Dependency
- Add code to the file
- Run a React application
Create a React application
Create a React app using the below command
npx create-react-app reactjs-file-upload-example
After running the above command system will create a new folder “reactjs-file-upload-example” so you need to move into that folder, use the below command to change the directory
cd reactjs-file-upload-example
Install Dependency
As you know we need to send data to the server, for this purpose we can use fetch or Axios, Axios is a promise-based HTTP client for Nodejs and browsers so we need to install this package.
npm install axios --save
Add code to the file
Create a component file to hold file upload code separate from another component and put the below code into that file
import React, { useState } from "react";
import axios from 'axios';
const FileUploadComponent = () => {
const [selectedFile, setSelectedFile] = useState("");
// On file select
let onFileChange = event => {
setSelectedFile(event.target.files[0]);
};
// File upload process
let onFileUpload = () => {
const formData = new FormData();
// Update the formData object
formData.append(
"myFile",
selectedFile,
selectedFile.name
);
axios.post("api/upload-image", formData)
.then((response) => {
// Success
console.log("File uploaded!");
})
.catch((error) => {
console.log(error);
});
};
let fileDetails = () => {
if (selectedFile) {
return (
<div>
<h2>File Details:</h2>
<p>File Name: {selectedFile.name}</p>
<p>File Type: {selectedFile.type}</p>
<p>File Size: {selectedFile.size}</p>
</div>
);
} else {
return (
<div>
<br />
<h4>Choose before Pressing the Upload button</h4>
</div>
);
}
};
return (
<>
<div>
<h1>
Technostuf.com
</h1>
<h3>
File Upload using ReactJS
</h3>
<div>
<input type="file" onChange={onFileChange} />
<button onClick={onFileUpload}>
Upload File
</button>
</div>
{fileDetails()}
</div>
</>
);
}
export default FileUploadComponent;
After adding a code, you have called this file upload component file into your code, in my case I am adding it to App.js
import './App.css';
import FileUploadComponent from './FileUploadComponent';
function App() {
return (
<div className="App">
<FileUploadComponent />
</div>
);
}
export default App;
Run a React application
Run the React application using the following command
npm start
After compilation, the program opens your browser and runs http://localhost:3000/
You can download the working example of a Reactjs file upload example Tutorial
https://github.com/technostuf/reactjs-file-upload-example
See Result
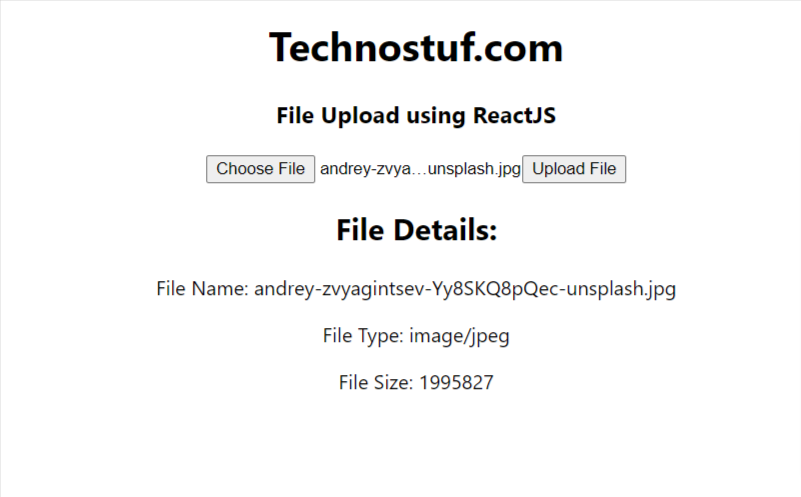
Related Post
- React Material UI Form example
- React Material UI Autocomplete with getting selected value
- React Area chart using recharts example
- React Pie chart using recharts with legend and custom label
- React google maps draggable marker example
- React datepicker using the most popular react-datepicker library
- React toast notification using react-toastify with example
- React responsive carousel slider with react-slick
- React tooltip using rc-tooltip with example
- React hook form schema validation using yup